Authentication
Introduction
In order to login and begin interacting with the API, you will need generate an access token using your username and password. The process follows the resource owner password flow defined in OAuth2.
- The client sends credentials to the authorization server.
- The authorization server authenticates the credentials and returns an access token.
- To access a protected resource, the client includes the access token in the Authorization header of the HTTP request.
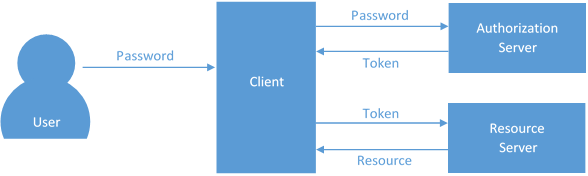
Example
$.ajax({ type: 'POST', url: '/account/login', data: { grant_type: 'password', username: 'username', password: 'password' } }).done(function (data) { var user = { id: data.id, username: data.userName, firstName: data.firstName, lastName: data.lastName } // Cache the access token in session storage. sessionStorage.setItem(tokenKey, data.access_token); }).fail(showError);
In the example above, we post the username, password and grant type to the login page. The grant type will always be ‘password’ due to the way the system authenticates. The code should create a request similar to the following;
POST https://ofd-api.blueprintcpq.net/account/login HTTP/1.1 Host: ofd-api.blueprintcpq.net:443 User-Agent: Mozilla/5.0 (Windows NT 6.3; WOW64; rv:32.0) Gecko/20100101 Firefox/32.0 Accept: */* Content-Type: application/x-www-form-urlencoded; charset=UTF-8 X-Requested-With: XMLHttpRequest Referer: https://example.blueprintcpq.net/ Content-Length: 55 grant_type=password&username=username&password=password
Assuming that the request is valid, you will receive a response containing a JSON payload that contains the users Id, username, first and last name, as well as an access token, when the token was issued and when it will expire. You should now store the token for future requests until the token expires. An example of the response is as follows;
HTTP/1.1 200 OK Content-Length: 669 Content-Type: application/json;charset=UTF-8 Server: Microsoft-IIS/8.0 Date: Wed, 01 Oct 2014 01:22:36 GMT { "access_token":"imSXTs2OqSrGWzsFQhIXziFCO3rF...", "token_type":"bearer", "expires_in":1209599, "id":"c8dcd36f-8f07-5e20-a8cd-8b72051bc374", "userName":"username", "firstName":"First", "lastName":"Last", ".issued":"Wed, 01 Oct 2014 01:22:33 GMT", ".expires":"Wed, 15 Oct 2014 01:22:33 GMT" }
Future Requests
In any future requests, you will need to send the access token along with the rest of the request. This is done by adding an authorization header. The authorization method to use is Bearer. The following is an example request with an authorization header added;
GET https://ofd-api.blueprintcpq.net/pages HTTP/1.1 Host: ofd-api.blueprintcpq.net:443 User-Agent: Mozilla/5.0 (Windows NT 6.3; WOW64; rv:32.0) Gecko/20100101 Firefox/32.0 Accept: */* Authorization: Bearer imSXTs2OqSrGWzsFQhIXziFCO3rF... X-Requested-With: XMLHttpRequest
If you try to access the API without an authorization header (or invalid), you will receive a 401 unauthorized response.